Installing a Specific Package Version with pip
Imagine your Python environment as a toolbox—just like you need the right tools for specific tasks, you require precise packages in the correct sizes and versions to tackle different programming challenges.
Just as you wouldn't use a hammer for every job, you need specific versions of Python packages customized to your project's requirements. However, traversing the complexities of package versions can often feel like searching through a messy toolbox.
So, here's the deal: as Python developers, we often need to install specific versions of packages for our projects. It's like trying to fit puzzle pieces together—if the pieces don't match, things just won't work smoothly. Maybe you've tried newer versions of a package, and things just aren't clicking, or you're trying to keep things consistent across different setups. The struggle is real.
The good news is that pip
, is Python's package installer. With pip
, you can say, "Hey, I want exactly this version of this package, please!". In this blog post, we'll learn how to use pip
effectively to ensure your Python projects always work smoothly. Let's get this toolbox organized, shall we?
- Overview of Version Numbers in Python Packages
- pip Command and its Options for Installing Packages
- Installing specific package versions with pip
- Managing Package Versions
- Managing Python Package Versions with Virtual Environments
Overview of Version Numbers in Python Packages
In Python packages, version numbers are important in indicating the specific release or iteration of the package. These version numbers follow a standard format known as semantic versioning (SemVer).
Semantic versioning consists of three parts: major, minor, and patch versions.
- The major version indicates significant changes that may not be backward compatible with previous versions.
- The minor version denotes added functionality in a backward-compatible manner.
- The patch version signifies bug fixes or minor updates that maintain backward compatibility.
Example:
version number like 1.2.3:
- 1 is the major version
- 2 is the minor version
- 3 is the patch version
Understanding version numbers is important because it helps you to track changes, manage dependencies, and ensure compatibility with their projects. By paying attention to version numbers, developers can decide when to upgrade or stick with a specific package version.
pip Command and its Options for Installing Packages
Here are some useful options for pip
when installing packages:
What is a pip?
pip
is the package installer for Python. You can install and manage additional libraries and dependencies that are not included in the standard Python library.
Before we jump into installing specific package versions with pip
, let's take a quick look at all the things we can do with pip
! We'll explore the different options and flags available for installing packages.
--version
: Specify the desired version of a package to install.--force-reinstall
: Reinstall a package, even if it's already installed.--no-deps
: Skip installing package dependencies.--index-url
: Use a custom package index URL for installation.--find-links
: Search for packages from local or remote locations.--proxy
: Set the proxy server to use during installation.--no-cache-dir
: Disable the cache directory for package downloads.--target
: Install packages into a specific directory instead of the default Python environment.
Installing Specific Package Versions with pip
When installing specific package versions with pip
, you can use various operators to specify the version range or exact version of the package you want to install. Explanation of each operator, along with examples and expected outputs:
1. Exact Version Match (==):
It installs the exact version of the requests package.
Syntax:
pip install package_name==version
Example:
pip install requests==2.25.1
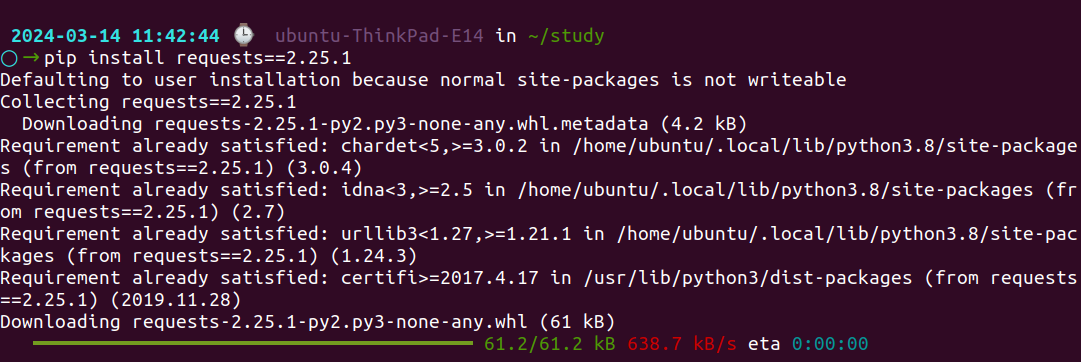
2. Greater Than (>):
It installs the latest version of the package that is greater than version given.
Syntax:
pip install package_name>version
Example:
pip install numpy>1.20.0
3. Less Than (<):
It installs the latest version of the package that is less than the version specified.
Syntax:
pip install package_name<version
Example:
pip install scipy<1.6.0
4. Greater Than or Equal To (>=):
It installs the latest version of the package that is greater than or equal to version given.
Syntax:
pip install package_name>=version
Example:
pip install matplotlib>=version
5. Less Than or Equal To (<=):
It installs the latest version of the package that is less than or equal to the version given.
Syntax:
pip install package_name<=version
Example:
pip install matplotlib<=3.4.3
6. Range (,):
It installs the latest version of the package that is greater than or equal to the version of the lower bound but less than version of the upper bound.
Syntax:
pip install package_name>=lower_bound,<upper_bound
Example:
pip install tensorflow>=2.0.0,<3.0.0
7. Range Inclusive ([,]):
It installs the latest version of the package that is greater than or equal to the version of a lower bound, including a version of the upper bound if available.
Syntax:
pip install package_name>=lower_bound,[upper_bound]
Example:
pip install scikit-learn>=0.24.0,[0.24.2]
8. Compatible Release (~=):
It installs the latest version of the package that is compatible with version specified, which allows minor updates but keeps the major version fixed.
Syntax:
pip install package_name~=version
Example:
pip install numpy~=1.21.0
Managing Package Versions
Considerations for upgrading and downgrading package versions while maintaining project stability involve careful planning and testing to ensure that changes in package versions do not introduce unexpected issues or break existing functionality in your project.
Upgrading Package Versions:
When we want to stay up-to-date and make the most of the latest and greatest features, bug fixes, and improvements, we use this --upgrade
flag.
Consider using virtual environments or dependency management tools like pipenv
or conda
to isolate package upgrades from other projects.
Example:
pip install --upgrade package_name
Downgrading Package Versions:
When newer versions cause problems or break existing functionality, we may need to revert to a previous version for stability, addressing compatibility issues or bugs
Test the downgraded version in a development or staging environment to ensure that it resolves the issues encountered with the newer version.
Example:
pip install package_name==desired_version
Important notes
Keep in mind that if the package version you're specifying isn't available on the Python Package Index (PyPI), you'll encounter an error.
Let's say you're working on a Python project, and you want to install a specific version of the requests
package, version 3.13. However, version 3.13 hasn't been released yet on PyPI. When you try to install it using pip
:
pip install requests==3.13
You'll encounter an error:
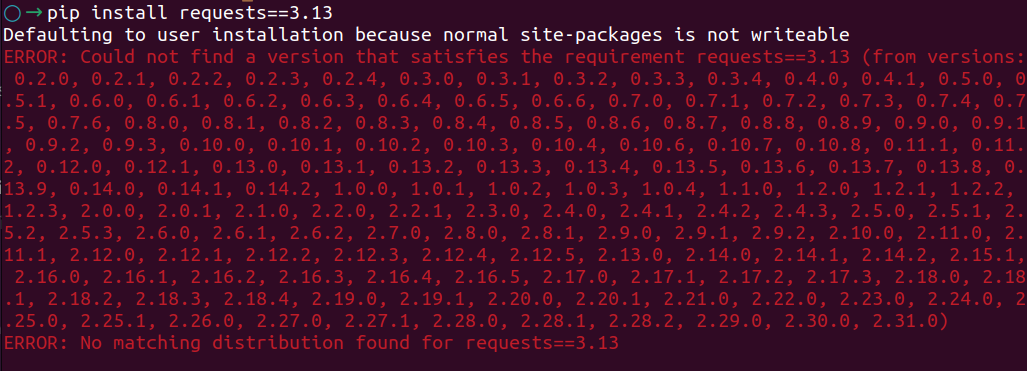
This error occurs because version 3.13 of the requests
package hasn't been uploaded to PyPI yet. In this case, you'll need to either use an available version of the package or wait until version 3.13 is released and uploaded to PyPI.
Managing Python Package Versions with Virtual Environments
Have you ever wondered how to install an older version of a Python package using PIP? What if you already have a different version installed? It can be tricky to manage different versions of packages without causing conflicts or breaking things.
The solution lies in using virtual environments! Instead of installing packages globally, you can create a virtual environment for each project. This way, you can freely install specific versions of packages without worrying about conflicts with other projects or the system-wide installation.
Virtual environments act like isolated bubbles where you can experiment with different package versions without affecting anything else. So, next time you need to install an older version of a Python package, create a virtual environment and install the version you need without any worries!
To conclude, understanding how to use pip
to install specific package versions and leverage virtual environments is essential for any Python developer. These tools ensure that your projects remain conflict-free and manageable, allowing flexibility and stability in your development workflow.
Atatus: Python Performance Monitoring
Atatus is an Application Performance Management (APM) solution that collects all requests to your Python applications without requiring you to change your source code. However, the tool does more than just keep track of your application's performance.
Monitor logs from all of your Python applications and systems into a centralized and easy-to-navigate user interface, allowing you to troubleshoot faster using Python monitoring.
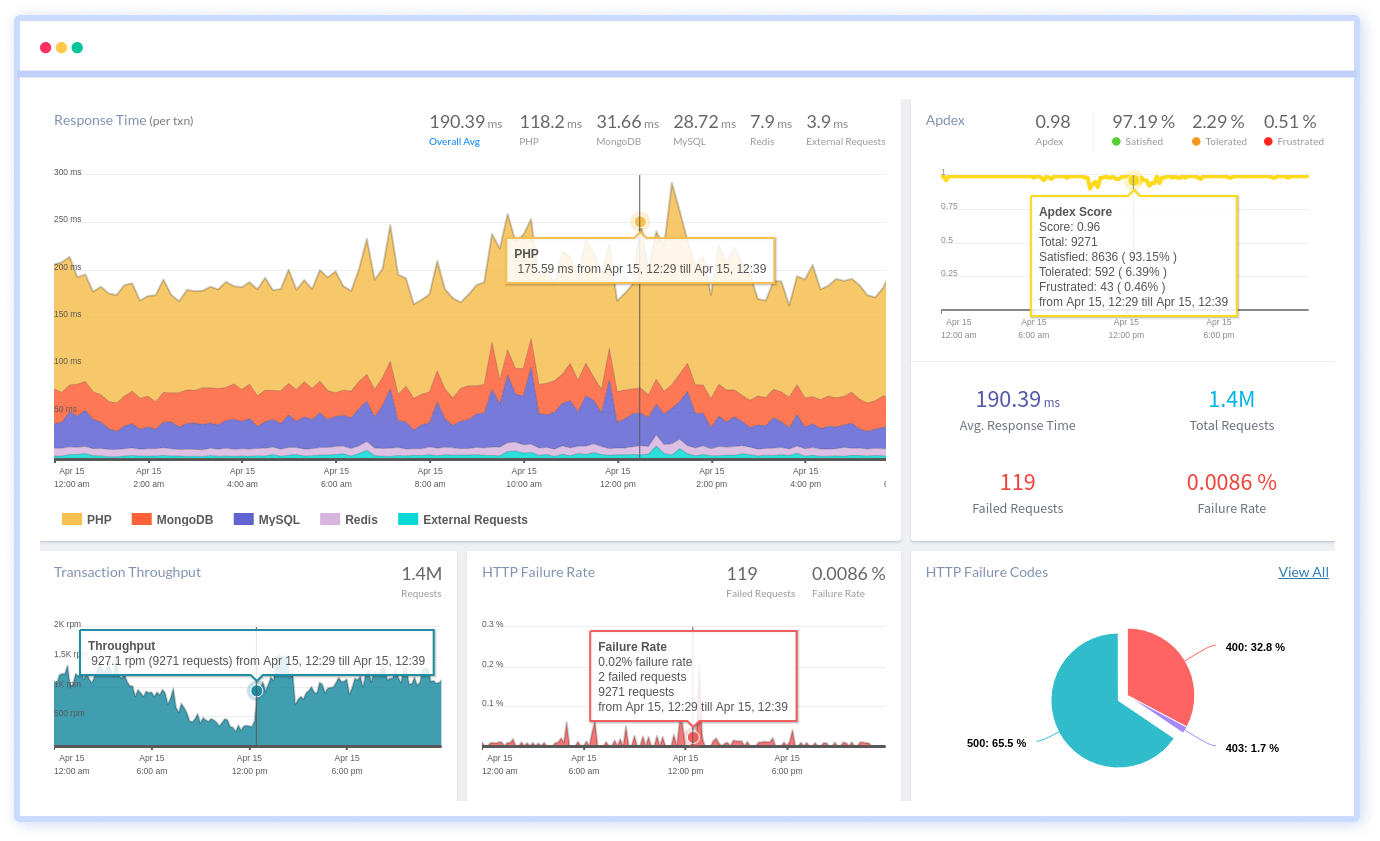
We give a cost-effective, scalable method to centralized Python logging, so you can obtain total insight across your complex architecture. To cut through the noise and focus on the key events that matter, you can search the logs by hostname, service, source, messages, and more. When you can correlate log events with APM slow traces and errors, troubleshooting becomes easy.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More