How to Round Numbers in Python? Methods and Examples
Rounding numbers in Python is an important concept that simplifies calculations and improves the accuracy of results. It helps you adjust numbers by changing them to the nearest whole number or to a specific number of decimal places.
Whether you are working with financial data, scientific measurements, or just everyday calculations, rounding ensures that your results are easy to interpret and use. Python offers various ways to round numbers, from using its built-in round()
function to using libraries like math
, NumPy
, and Pandas
. Each method has its own approach to rounding.
This blog explores the different methods and functions available in Python to handle rounding and explains how to apply them in your code effectively.
- What does rounding a number in python mean?
- Python’s built-in round() function
- Different ways to round a number in python
- Round numbers using math library
- Round numbers using truncation concept
- Round numbers using rounding bias concept
- Using other python libraries for rounding
What does rounding a number in python mean?
Rounding a number in python means changing it to the nearest whole number or to a certain number of decimal places. This helps make numbers easier to use or more accurate. Rounding numbers plays an important role in various programming contexts, as it helps simplify data, improve performance, and ensure consistency in calculations.
Python’s built-in round() function
The round()
function is a built-in python function that takes two numeric arguments and rounds a number to the specified number of decimal places.
Syntax:
round(number, ndigits)
number
parameter is the numeric value you want to round. It can be either an integer (whole number) or a floating-point number (decimal).ndigits
is an optional parameter that specifies the number of decimal places to round the number to. The default value is 0.
For example, to round a number to two decimal places in python, the round()
function is the easiest way to get a specific number of decimal places. lets see a basic example of how to use the round()
function in python.
number = 5.2134
rounded_number = round(number, 2)
print(rounded_number)
Output:

Different ways to round a number in python
Rounding in Python can be done in various ways to simplify or adjust numeric values. You can round numbers to the nearest integer or to a specific number of decimal places depending on your needs.
Round to the nearest integer
This is the simplest form of rounding, where the number is rounded to the closest whole number. The round()
function, when used without a second parameter, rounds the number to the nearest whole number.
print(round(4.6))
print(round(4.2))
Output:

In this example, the round()
function rounds the variable numbers 4.6
and 4.2
to the nearest integers which are 5
and 4
in this case.
Round to a specific decimal place
Rounding to a specific decimal place means you can round a number to a set number of decimal places by passing a second parameter ndigits
with round()
, which specifies how many decimals you want.
print(round(3.14159, 2))
print(round(2.71828, 3))
Output:

In this example, the round()
function rounds the numbers 3.14159
and 2.71828
based on the second parameter to two and three decimal places, respectively. This results in 3.14
for the first number and 2.718
for the second.
Round numbers using math library
The math library in python provides the ceil()
and floor()
functions to round numbers up and down to the nearest integer, respectively.
To round a number up to the nearest integer, you can use the math.ceil()
function from python's built-in math
module. It ensures the number is always rounded up, regardless of any decimal value.
Here’s an example:
import math
number = 5.24
rounded_number = math.ceil(number)
print(rounded_number)
Output:

In this case, the math.ceil()
function will round the number 5.24 up to the nearest integer, which is 6
.
To round a number down to the nearest integer in python, you can use the math.floor()
function from the built-in math
module. This function always rounds down, regardless of the decimal value.
Here’s an example:
import math
number = 7.89
rounded_number = math.floor(number)
print(rounded_number)
Output:
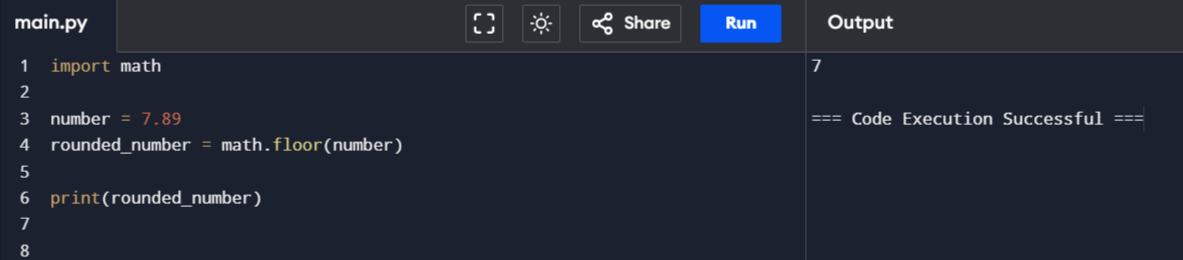
In this output, the math.floor()
function rounds the number 7.89
down to the nearest integer, which is 7
.
Round numbers using truncation concept
The concept of rounding numbers using truncation involves cutting off the decimal part of a number, without rounding, and leaving just the integer part. However, you can also extend this concept by replacing digits after a given position with 0
. The truncate()
function from python’s math
module can be used for this purpose, and it works for both positive and negative numbers.
(i). Basic truncation (removing decimal part)
When we talk about truncation, it typically refers to simply removing the decimal part of a number. This can be done using either the int()
function or math.trunc()
.
Code Example:
import math
# Positive number
print(math.trunc(5.78))
# negative number
print(math.trunc(-5.78))
Output:

(ii). Truncating and replacing digits with 0
In some cases, you may want to replace the digits after a certain position with 0, similar to rounding but without adjusting the value up or down.
Lets see a code example to understand this,
import math
def truncate_with_zero(number, position):
multiplier = 10 ** position
truncated_number = math.trunc(number / multiplier) * multiplier
return truncated_number
print(truncate_with_zero(5678, 2))
print(truncate_with_zero(-5678, 3))
Output:
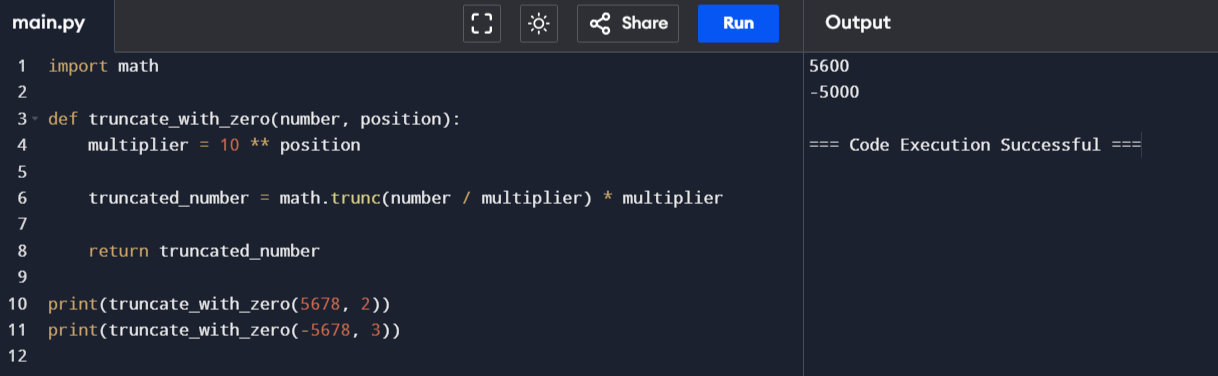
Round numbers using rounding bias concept
Rounding up pushes values toward positive infinity (up). For example, if you have 3.1
, it gets rounded to 4
. This creates a positive bias because numbers tend to get bigger. On the other hand, rounding down pushes values toward negative infinity (down). For example, 3.9
would become 3
, and this creates a negative bias because numbers tend to get smaller.
Similarly, truncation pulls positive numbers down and negative numbers up. If you have 5.9
and -5.9
, truncation cuts off the decimals, giving 5
for positive and -5
for negative, pulling them both toward zero. This method creates a zero bias because it pulls positive numbers down and pushes negative numbers up.
(i). Rounding half up
Round up
always rounds to the next highest value, no matter how close the number is to the lower value. On the other hand, Round half up
addresses the common need to round numbers to the nearest value. It specifically rounds up when a number is exactly halfway between two options, which avoids bias in rounding.
import math
def round_half_up(n, decimals=0):
multiplier = 10 ** decimals
return math.floor(n * multiplier + 0.5) / multiplier
print(round_half_up(2.34, 1))
print(round_half_up(-2.5))
print(round_half_up(-1.678, 2))
print(round_half_up(3.555, 2))
Output:
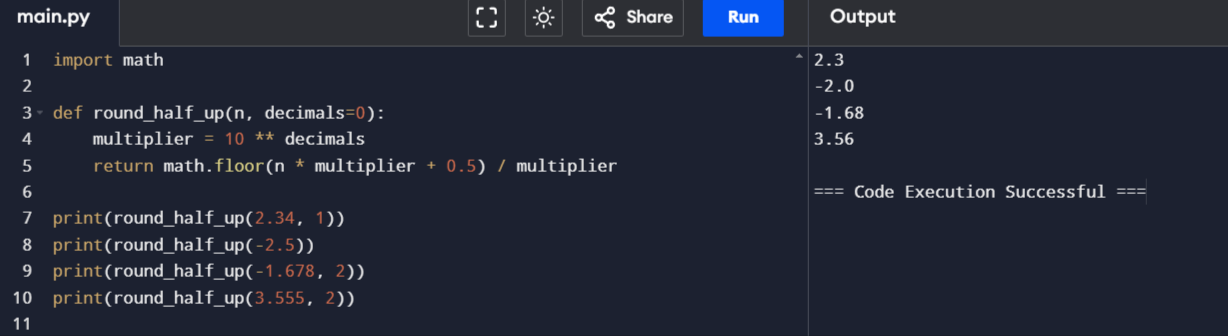
(ii). Rounding half down
Similarly, Round half down
is a rounding method that rounds numbers to the nearest value, but when a number is exactly halfway between two options, it rounds down to the lower value instead of rounding up.
import math
def round_half_down(n, decimals=0):
multiplier = 10 ** decimals
return math.floor(n * multiplier - 0.5) / multiplier
print("Round Half Down Results:")
print(round_half_down(2.4,1))
print(round_half_down(3.5))
print(round_half_down(5.75, 1))
print(round_half_down(-1.5))
Output:
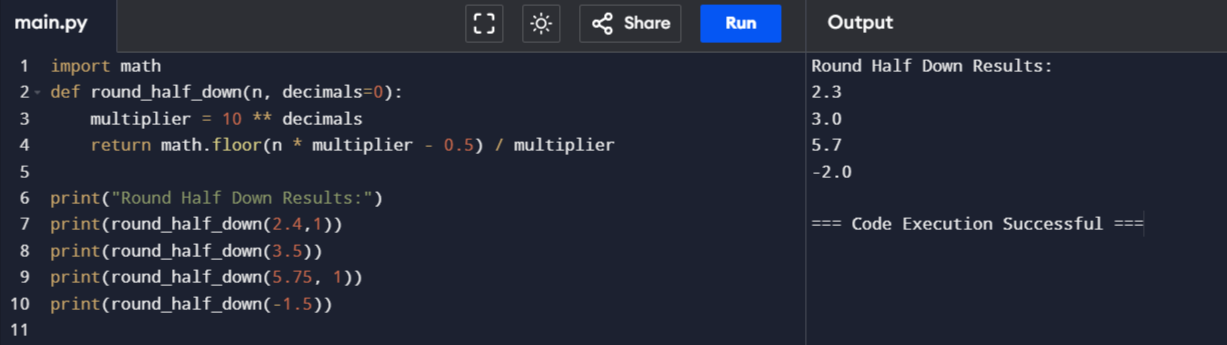
(iii). Rounding half away from zero
This method rounds numbers based on the value of the digit immediately after the rounding point. There are four cases to consider when rounding:
- If n is positive and d >= 5: Round up to the next whole number.
- If n is positive and d < 5: Round down to the nearest whole number.
- If n is negative and d >= 5: Round down.
- If n is negative and d < 5: Round up (away from zero).
[ where n= The number you want to round and d= The digit immediately after the rounding point (the decimal place) ]
import math
def round_half_away_from_zero(n):
if n > 0:
return math.floor(n + 0.5)
else:
return math.ceil(n - 0.5)
print(round_half_away_from_zero(2.5))
print(round_half_away_from_zero(2.4))
print(round_half_away_from_zero(-2.5))
print(round_half_away_from_zero(-2.4))
Output:
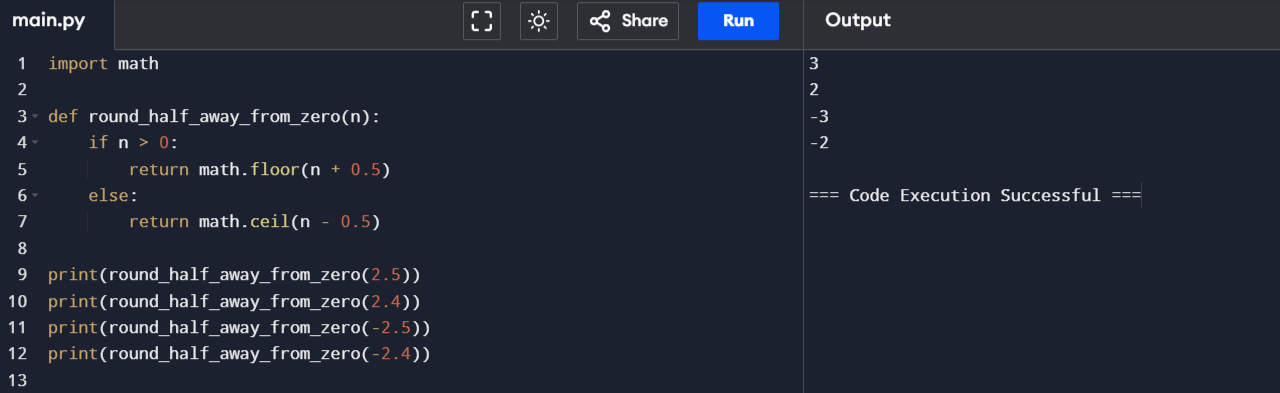
Using other python libraries for rounding
(i). numpy.round_() in python
NumPy is a widely used library in python that allows for rounding arrays to a specified number of decimal places. It operates similarly to python’s built-in round()
function but can handle array-like inputs.
import numpy as np
arr = np.array([1.678, 2.123, 3.456])
rounded_arr = np.around(arr, decimals=2)
print(rounded_arr)
Output:
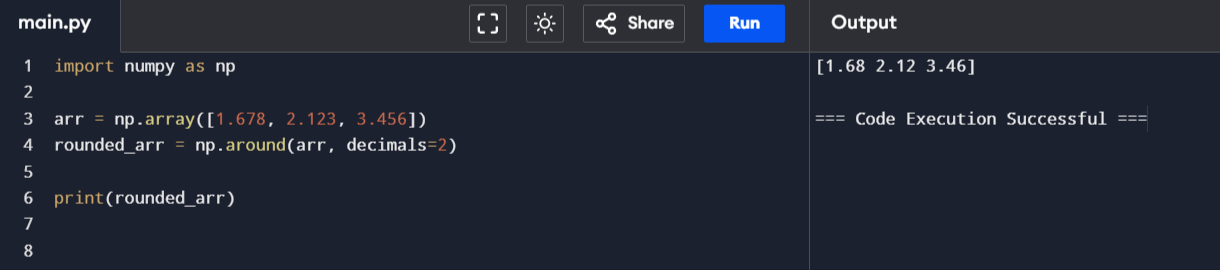
(ii). Pandas DataFrame round() in python
Pandas, a popular library in python, provides a convenient way to perform rounding operations on DataFrame columns using the round()
method. This method allows you to round the values in one or more columns to a specified number of decimal places.
import pandas as pd
data = {'values': [1.234, 8.654, 4.321]}
df = pd.DataFrame(data)
df['rounded_values'] = df['values'].round(decimals=1)
print(df)
Output:
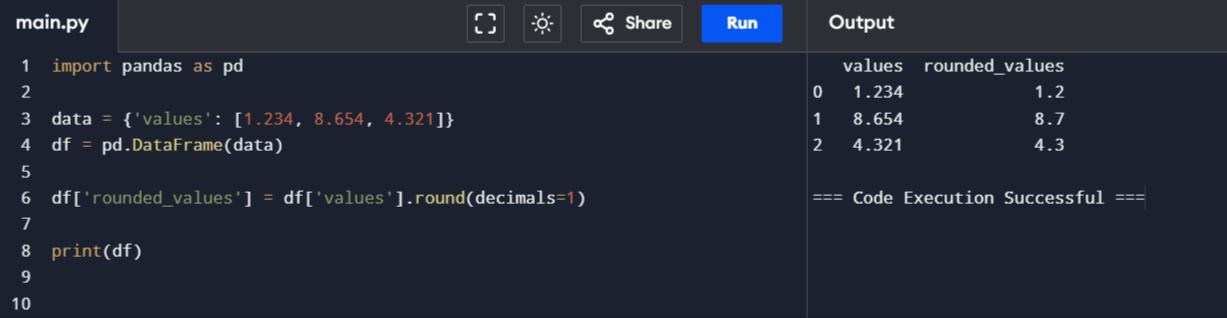
Conclusion
Rounding numbers in Python is an essential tool for simplifying data and ensuring precision in various applications. Whether you are using the basic round()
function or exploring advanced methods with libraries like math
, NumPy
, and Pandas
, Python provides versatile options to meet your needs.
By choosing the right rounding method, you can handle numbers efficiently and make your calculations clearer and more accurate. Understanding these techniques will help you confidently work with numbers in coding.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More